Instagram Graph API
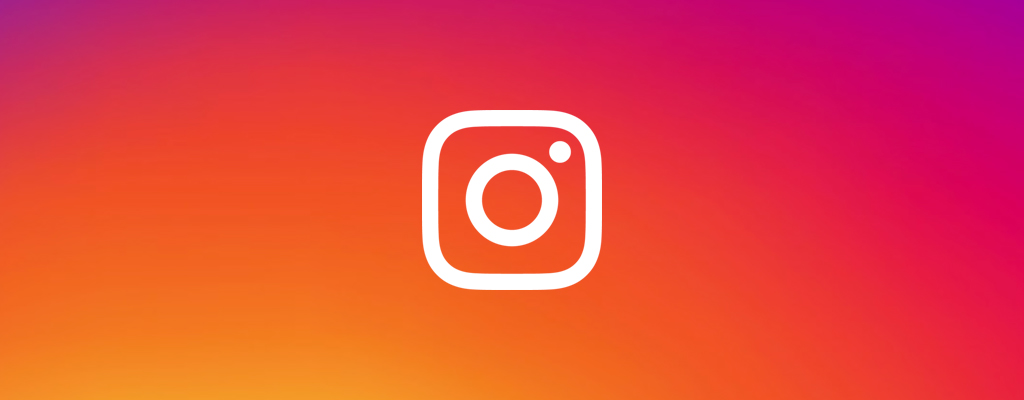
前回は、Instagram Graph APIが使えるようになるまでの設定方法等を書きました。
今回は、Instagram Graph APIを使用してInstagramの写真をWebサイトに表示するためのプログラムを書いてみます。
サーバサイドを実装
JavaScriptだけでも表示することはできるけど、アクセストークンが簡単にバレてしまうので、今回はサーバーサイドプログラム(PHP)も組み合わせた形で開発します。
前回の設定で、自分の投稿のみにアクセスできれば良い設定にしたので、それに沿ったプログラムにします。
PHP
今回は、cURL関数を使用します。
【】内の値は、前回取得した指定された値に置き換えます。
instagram.api.php
<?php
$instagram_api_url = 'https://graph.facebook.com/v5.0/';
$instagram_business_id = '【InstagramビジネスアカウントID】';
$access_token = '【3段階目のアクセストークン】';
$query = 'name,media{caption,like_count,media_url,permalink,timestamp,username}&access_token='. $access_token;
$url = $instagram_api_url . $instagram_business_id .'?fields='. $query;
$ch = curl_init();
curl_setopt( $ch, CURLOPT_URL, $url );
curl_setopt( $ch, CURLOPT_RETURNTRANSFER, true );
$data = curl_exec($ch);
curl_close($ch);
echo $data;
exit;
?>
フロントサイドを実装
HTML
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<title>Instagram Graph API Sample</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<ul id="instagram-photo"></ul>
<script src="instagram.js"></script>
</body>
</html>
index.html
JavaScript
先ほど作ったinstagram.api.phpからJSON形式でデータを受け取ります。
※ES6やjQueryなど、色んな書き方があると思うけど、今回は、ピュアなJavaScriptにしました。
instagram.js
( function()
{
var api = './instagram.api.php';
var request = new XMLHttpRequest();
request.open( 'GET', api, true );
request.responseType = 'json';
request.onload = function( e )
{
if( request.readyState === 4 ){
if( request.status === 200 ){
var data = request.response.media.data;
var fragment = document.createDocumentFragment();
data.forEach( function( photo, idx )
{
var item = document.createElement('li');
var img = document.createElement('img');
item.setAttribute( 'class', 'instagram-photo-item' );
img.setAttribute( 'src', photo.media_url );
item.appendChild(img);
fragment.appendChild(item);
});
document.getElementById('instagram-photo').appendChild(fragment);
}else{
console.log(request.statusText);
}
}
};
request.onerror = function( e )
{
console.log(e.type);
};
request.send(null);
})();
CSS
本当に表示されればそれで良し。程度だけど・・・
style.css
#instagram-photo {
display: flex;
flex-wrap: wrap;
}
.instagram-photo-item {
width: 25%;
padding: 1em;
}
img {
max-width: 100%;
height: auto;
}
CSS自体は内容が全く異なるけど、このプログラムを拡張しつつInstagramの写真を当サイトのトップページに表示させてます。表示サンプル